Higher-Order Components
Pass reusable logic down as props to components throughout your application
#Overview
Higher-Order Components (HOC) make it easy to pass logic to components by wrapping them.
For example, if we wanted to easily change the styles of a text by making the font larger and the font weight bolder, we could create two Higher-Order Components:
withLargeFontSize
, which appends thefont-size: "90px"
field to thestyle
attribute.withBoldFontWeight
, which appends thefont-weight: "bold"
field to thestyle
attribute.
Any component that's wrapped with either of these higher-order components will get a larger font size, a bolder font weight, or both!
#Implementation
We can apply logic to another component, by:
- Receiving another component as its
props
- Applying additional logic to the passed component
- Returning the same or a new component with additional logic
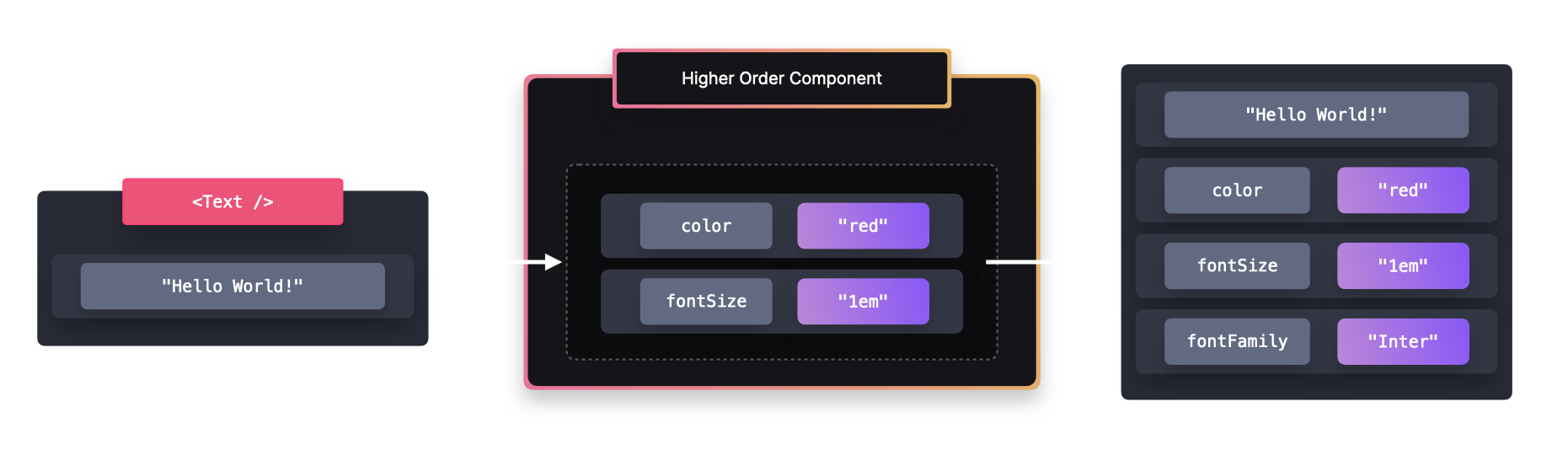
To implement the above example, we can create a withStyles
HOC that adds a color
and font-size
prop to the component's style.
function withStyles(Component) {
return (props) => {
const style = {
color: 'red',
fontSize: '1em',
// Merge props
...props.style,
};
return <Component {...props} style={style} />;
};
}
const Text = ({ style }) => <p style={style}>Higher Order Component</p>;
const StyledText = withStyles(Text);
jsx
Note
If you have a component that always needs to be wrapped within a HOC, you can also directly pass it instead of creating two separate components like we did in the example above.
const Text = withStyles(() => <p style={{ fontFamily: 'Inter' }}>Hello world!</p>);
js